7.2 在PHP中操作数据库
通过PHP是可以访问及操作MySQL的,本节将介绍如何使用PHP访问与操作MySQL。
7.2.1 连接到一个MySQL数据库
访问并处理数据库中的数据之前,必须创建到达数据库的连接。在PHP中,可以通过使用mysql_connect()函数完成。
语法:
mysql_connect(servername,username,password);
其中:
servername:可选,规定要连接的服务器。默认是“localhost”。
username:可选,规定登录所使用的用户名。默认值是拥有服务器进程的用户的名称。
password:可选,规定登录所用的密码。默认是“”。
若要关闭连接,可以使用mysql_close()函数。
7.2.2 访问数据库中的数据
在PHP中,使用mysql_query()函数来执行select语句。函数语法如下所示。
mysql_query(query,connection)
其中,query参数必需,规定要发送的SQL查询。注意:查询字符串不应以分号结束。connection参数可选。规定SQL连接标识符。如果未规定,则使用上一个打开的连接。
如果没有打开的连接,本函数会尝试无参数调用mysql_connect()函数来建立一个连接并使用它。返回值mysql_query()仅对SELECT,SHOW,EXPLAIN或DESCRIBE语句返回一个资源标识符,如果查询执行不正确则返回false。对于其他类型的SQL语句,mysql_query()在执行成功时返回true,出错时返回false。非false的返回值意味着查询是合法的并能够被服务器执行。这并不说明任何有关影响到的或返回的行数。很有可能一条查询执行成功了但并未影响到或并未返回任何行。7-1.php实现在Web页面中显示tstudent表中的所有记录。
7-1.php
<?php $connect = mysql_connect('localhost', 'root', '') or die('Could not connect: ' . mysql_error());//使用mysql_connect()函数创建数据库连接, 若数据连接创建失败,就会运行or后面的die()函数,终止程序并显示“无法创建连接” mysql_query("SET NAMES 'GB2312'",$connect);// 执行一条SQL查询,本语 句设置查询所使用的字符集 echo '<b>第一步:</b>成功建立连接! <BR>'; $db = 'hbsi_school'; mysql_select_db($db) or die('Could not select database ('.$db.') because of : '.mysql_error());//使用mysql_connect()函数打开数据库, 若数据库打开失败,就会运行or后面的die()函数,终止程序并显示相关提示信息 echo '<b>第二步:</b> 成功连接到 ('.$db.') !<BR>'; $query = 'SELECT * FROM tstudent'; $result = mysql_query($query) or die('Query failed: ' . mysql_ error());//mysql_query() 函数执行一条 MySQL 查询 echo "<b>获取数据...</b><br/>"; $tableresult="<table border='1' width='1000'>"; $count = 0; while ($line = mysql_fetch_array($result, MYSQL_ASSOC))/ *mysql_ fetch_array() 函数从结果集中取得一行作为关联数组,或数字数组,或二者兼有 返回根据从结果集取得的行生成的数组,如果没有更多行则返回 false。*/ { $sid=$line["sid"]; $tableresult.= "<tr>"; foreach ($line as $col_value) { $tableresult.="<td>$col_value</td>"; } $count++; $tableresult.="</tr>"; } $tableresult.="</table>"; if ($count < 1) { echo "<br><br>表格中未发现记录.<br><br>"; } else { echo $tableresult; echo "<br/><h1>表格中有".$count."行记录.</h1><br/>"; } mysql_free_result($result); mysql_close($connect); ?> </body> </html>
运行效果如图7-21所示。
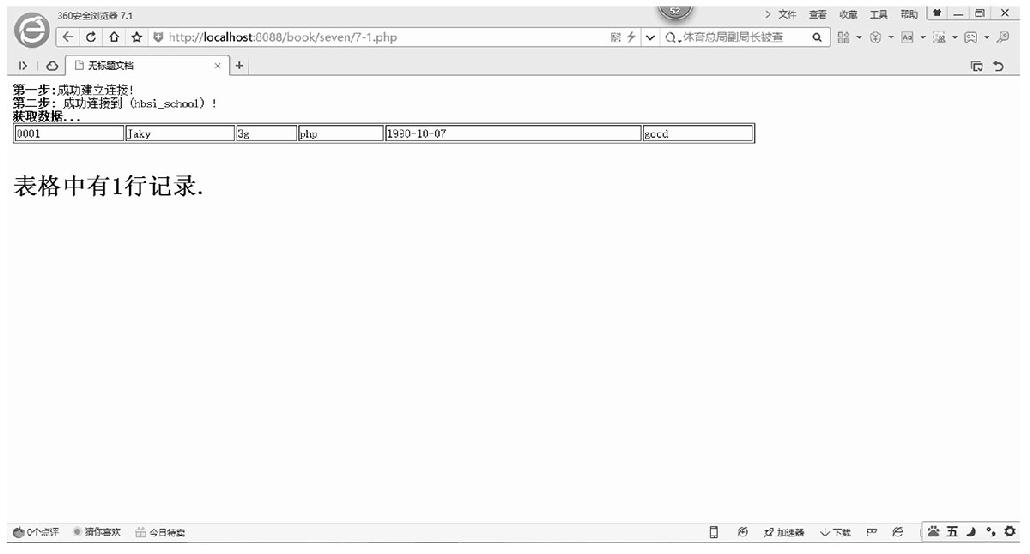
图7-21 查询表中数据运行效果图
7.2.3 插入、修改、删除记录
在PHP中插入、修改、删除记录同样使用mysql_query()函数来实现。如果插入、修改、删除操作执行成功函数返回值为true,出错时返回false。修改表tstudent内容的示例如7-2.php所示。
7-2.php
<html> <head> <meta http-equiv="Content-Type" content="text/html; charset=gb2312"> <title>无标题文档</title> </head> <body> <?php $connection=mysql_connect('localhost','root',''); mysql_query("SET NAMES 'GB2312'",$connection); $db="hbsi_school"; mysql_select_db($db) or die('Could not select database ('.$db.') because of : '.mysql_error()); $sql="update tstudent set sname='xyq' "." where sid='".$sid."'"; $result=mysql_query($sql); if ($result) { echo "<h1>update success</h1>"; } else { echo "<h1>update defeat</h1>".mysql_error(); } ?> </body> </html>
通过PHP向表中插入记录,示例如7-3.php所示。
7-3.php
<html> <head> <meta http-equiv="Content-Type" content="text/html; charset=gb2312"> <title>无标题文档</title> </head> <body> <?php $connection=mysql_connect('localhost','root',''); mysql_query("SET NAMES 'GB2312'",$connection); $db="hbsi_school"; mysql_select_db($db) or die('Could not select database ('.$db.') because of : '.mysql_error()); $sql="insert into tstudentvalues ('0002','jaky', 'male', 'software', '1980-02-02','good')"; $result=mysql_query($sql); if ($result) { echo "<h1>insert success</h1>"; } else { echo "<h1>insert defeat</h1>".mysql_error(); } ?> </body> </html>
通过PHP删除表中记录的示例如7-4.php所示。
7-4.php
<html> <head> <meta http-equiv="Content-Type" content="text/html; charset=gb2312"> <title>无标题文档</title> </head> <body> <?php $connection=mysql_connect('localhost','root',''); mysql_query("SET NAMES 'GB2312'",$connection); $db="hbsi_school"; mysql_select_db($db) or die('Could not select database ('.$db.') because of : '.mysql_error()); $sql="delete from tstudent where sid='0002'"; $result=mysql_query($sql); if ($result) { echo "<h1>delete success</h1>"; } else { echo "<h1>delete defeat</h1>"; } ?> </body> </html>
共有0条评论 网友评论
暂无评论,快来抢沙发吧!